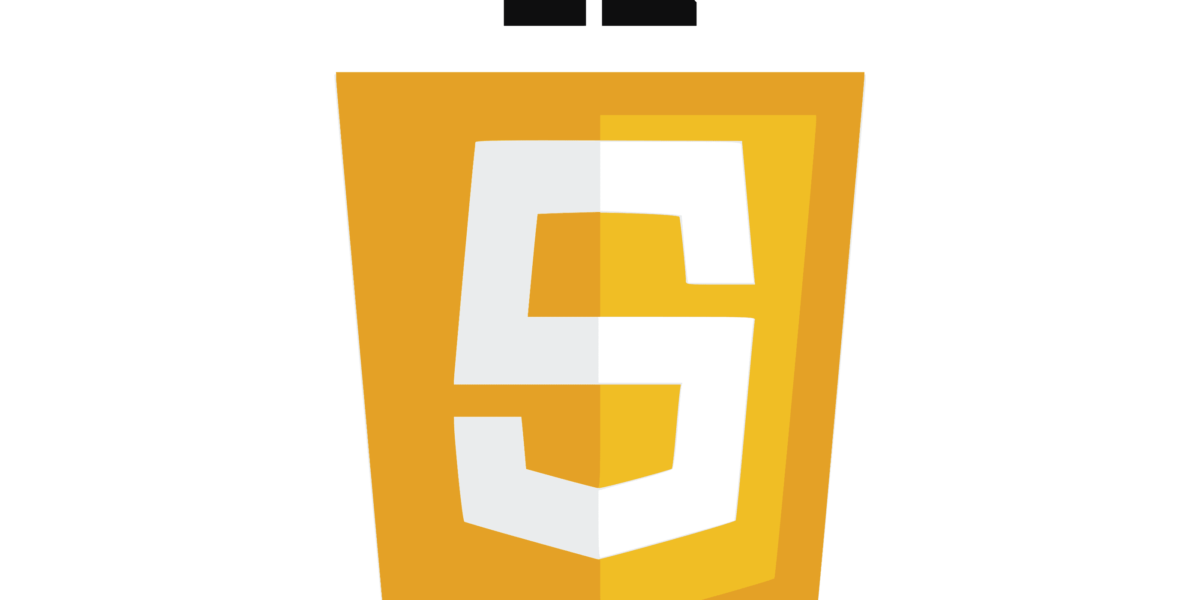
- Instructor: admin
- Lectures: 15
- Duration: 10 weeks
Categories: PROGRAMMING
For an online course service that offers JavaScript programming, we create a course that covers the following topics:
- Introduction to JavaScript: This section can cover the basics of JavaScript, including what it is, why it is important, and the different applications of JavaScript.
- JavaScript Language Fundamentals: In this section, you can discuss the basic concepts of JavaScript programming, including variables, data types, operators, and control structures.
- Functions and Objects: Here, you can cover JavaScript functions and objects, including how to create and use functions, how to define and manipulate objects, and how to use object-oriented programming concepts in JavaScript.
- DOM Manipulation: In this section, you can cover the Document Object Model (DOM) in JavaScript, including how to manipulate HTML elements using JavaScript, how to use events to trigger actions, and how to use AJAX to fetch data from the server.
- jQuery: Here, you can cover jQuery, a popular JavaScript library that simplifies DOM manipulation and event handling. You can cover how to use jQuery to create animations, manipulate styles, and handle events.
- React: In this section, you can provide an introduction to React, a popular JavaScript library for building user interfaces. You can cover how to create components, use state and props, and handle user events in React.
- Node.js: Here, you can cover Node.js, a JavaScript runtime that allows developers to run JavaScript on the server side. You can cover how to create a simple web server, use middleware, and work with databases.
- JavaScript Frameworks: Finally, you can provide an introduction to popular JavaScript frameworks such as Angular, Vue.js, and Ember.js, and how to use them for web application development.
To make the course engaging, we use a variety of teaching methods, including videos, lectures, quizzes, and programming exercises. We also offer a discussion forum where learners can ask questions and interact with their peers.
By offering a comprehensive JavaScript programming course, we provide learners with the skills they need to develop dynamic and interactive web applications using JavaScript. This is valuable for web developers, software engineers, and entrepreneurs who need to create custom web applications to meet their business needs.
-
Lessons
-
Lecture 1.1Introduction to JavaScript: history, uses, and applications
-
Lecture 1.2JavaScript syntax and data types
-
Lecture 1.3Variables, operators, and expressions
-
Lecture 1.4Control structures: if/else statements, loops, and switch statements
-
Lecture 1.5Functions: defining, calling, and passing arguments
-
Lecture 1.6Arrays and objects
-
Lecture 1.7DOM (Document Object Model) manipulation: selecting and modifying HTML elements using JavaScript
-
Lecture 1.8Event handling: adding interactivity to web pages with JavaScript
-
Lecture 1.9AJAX (Asynchronous JavaScript and XML): communicating with servers without reloading pages
-
Lecture 1.10jQuery: a JavaScript library for simplifying DOM manipulation and event handling
-
Lecture 1.11Frameworks and libraries: React, Angular, Vue.js, etc.
-
Lecture 1.12Debugging and error handling
-
Lecture 1.13Best practices and coding conventions
-
Lecture 1.14ES6 (ECMAScript 6) features and syntax, including let, const, arrow functions, and template literals
-
Lecture 1.15Web development tools and resources: IDEs, editors, debugging tools, and online resources for learning and practicing JavaScript.
-